What is jquery text() method?
It is used to change the entire text content of the selected elements.
text() method is used to set and also return the text content.
This is can be executed in three forms for the selected elements.
1. Retuning the existing text content.
2. Setting the new text content.
3. Calling function inside the method.
What is the syntax of text() method?
$(selector).text()
$(selector).text(content)
$(selector).text(function(index,currentcontent))
selector – this is the html element.
text() – this is an inbuilt jquery method
content – It is a mandatory parameter which specifies the new content to be replaced for the selected elements.
function(index, currentcontent) – It is an optional parameter which specifies a function returns the old/existing content for the selected elements.
index – This returns the index position of the element in the set.
currentcontent – It returns the current text content of the selected element.
What is the difference between html() and text() methods ?
html() method :
1. This will set or return the innerHTML(text in markup language) for the selected elements.
2. html() method can only used in html documents.
text() method :
1. This will set or return the actual text for the selected elements.
2. text() method can be used in both XML and HTML documents.
Jquery tutorial for beginners !!!
Click to Learn More about – online learn jquery
1. $(selector).text()
Example :
This empty text() method returns the existing/old content of the first matched element.
This alerts the existing text content.
<!DOCTYPE html>
<html>
<head>
<title>Our title</title>
<style>
#dId-1{
width: 30%;
height: 30%;
text-align:center;
padding: 20px;
margin: 10px;
border: 2px solid green;
font-size: 20px;
}
#dId-2{
width: 30%;
height: 30%;
text-align:center;
margin: 10px;
border: 2px solid green;
padding: 20px;
font-size: 20px;
}
.b1, .b2, .b3, .b4, .b5, .s1, .s2, .s3, .s4, .s5{
width:150px;
height:30px;
font-size: 20px;
}
</style>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.3.1/jquery.min.js">
</script>
<script>
$(document).ready(function(){
$(".b1").click(function(){
alert($(".p1").text());
});
$(".b2").click(function(){
alert($(".p2").text());
});
});
</script>
</head>
<body>
<div id="dId-1">
<p class="p1">This is Paragraph1 P1</p>
<p class="p2">This is Paragraph2 P2</p>
</div>
<div id="dId-2">
<p>
<button class="b1">Text</button> text() Method for P1
</p>
<p>
<button class="b2">Text</button> text() Method for P2
</p>
<div>
</body>
</html>
Output :
Getting existing content

2. $(selector).html(content)
This method is used to set text content for the selected elements.
Example :
<!DOCTYPE html>
<html>
<head>
<title>Our title</title>
<style>
#dId-1{
width: 30%;
height: 30%;
text-align:center;
padding: 20px;
margin: 10px;
border: 2px solid green;
font-size: 20px;
}
#dId-2{
width: 30%;
height: 30%;
text-align:center;
margin: 10px;
border: 2px solid green;
padding: 20px;
font-size: 20px;
}
.b1, .b2, .b3, .b4, .b5, .s1, .s2, .s3, .s4, .s5{
width:150px;
height:30px;
font-size: 20px;
}
</style>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.3.1/jquery.min.js">
</script>
<script>
$(document).ready(function(){
$(".b1").click(function(){
$(".p1").text("This is the new content replaced for P1");
});
$(".b2").click(function(){
$(".p2").text("This is the new content replaced for P2");
});
});
</script>
</head>
<body>
<div id="dId-1">
<p class="p1">This is Paragraph1 P1</p>
<p class="p2">This is Paragraph2 P2</p>
</div>
<div id="dId-2">
<p>
<button class="b1">Text</button> text() Method for P1
</p>
<p>
<button class="b2">Text</button> text() Method for P2
</p>
<div>
</body>
</html>
Output :
1. before calling text() method with P1 and P2 values.

2. after calling text() method setting new content for P1 and P2.

3 $(selector).text(function(index,currentcontent))
This is an optional parameter.
index – This returns the index position of the element in the set.
currentcontent – It returns the current text content of the selected element.
Example :
<!DOCTYPE html>
<html>
<head>
<title>Our title</title>
<style>
#dId-1{
width: 30%;
height: 30%;
text-align:center;
padding: 20px;
margin: 10px;
border: 2px solid green;
font-size: 20px;
}
#dId-2{
width: 30%;
height: 30%;
text-align:center;
margin: 10px;
border: 2px solid green;
padding: 20px;
font-size: 20px;
}
.b1, .b2, .b3, .b4, .b5, .s1, .s2, .s3, .s4, .s5{
width:150px;
height:30px;
font-size: 20px;
}
</style>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.3.1/jquery.min.js">
</script>
<script>
$(document).ready(function(){
$(".b1").click(function(){
$(".p1").text(function(n){
return alert($(".p1").text());
});
});
$(".b2").click(function(){
$(".p2").text(function(n){
return alert($(".p1").text());
});
});
});
</script>
</head>
<body>
<div id="dId-1">
<p class="p1">This is Paragraph1 P1</p>
<p class="p2">This is Paragraph2 P2</p>
</div>
<div id="dId-2">
<p>
<button class="b1">Text</button> text() Method for P1
</p>
<p>
<button class="b2">Text</button> text() Method for P2
</p>
<div>
</body>
</html>
Output :
1. Before calling text() method.
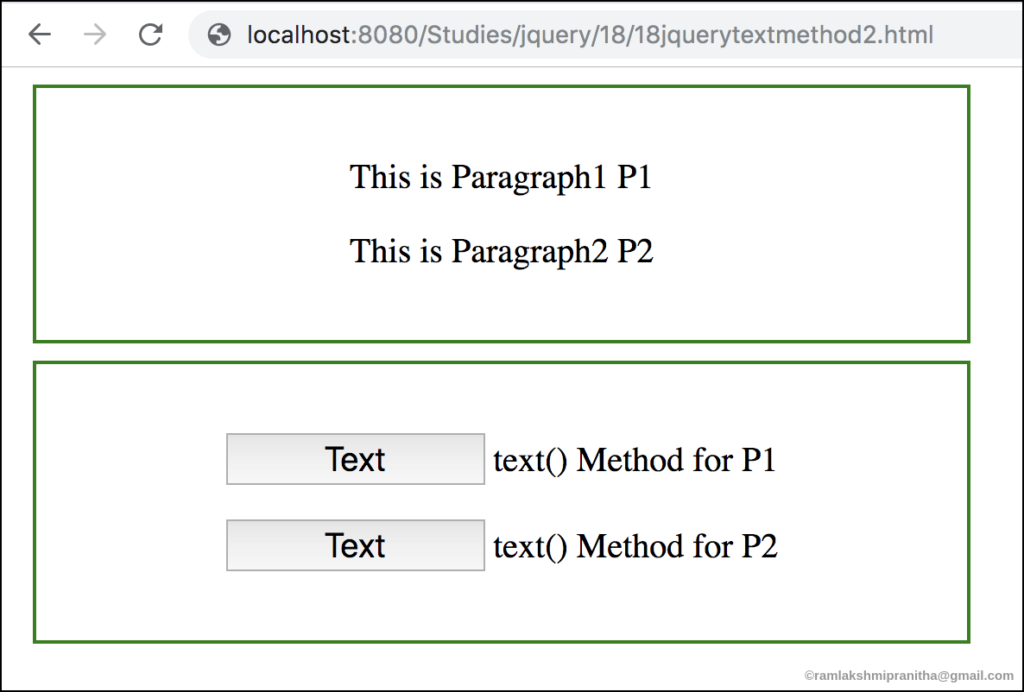
2. After calling text() method.
