What is jquery slideUp() method ?
JQuery inbuilt slideUp() method is used to slide-up the selected elements.
Elements visible are hidden in slideUp() method.
What is the syntax of slideUp() ?
$(selector).slideUp(speed,easing,callback)
selector – this is the html element.
slideUp() – inbuilt jquery method
There are 3 optional params for slideUp() method.
1. speed
2. easing
3. callback
1. speed
This defines the speed of the show element.
By default this will be 400 milliseconds.
Possible values are “milliseconds”,”slow”,”fast”.
2. easing
This defines the speed of the element at different point of animation.
By dafault the value is “swing”.
Possible values are “swing” and “linear”.
3. callback
This defines the call back function to be executed after show operation.
Jquery tutorial for beginners !!!
Click to Learn More about – online learn jquery
1. slideUp() method called.
Example :
<!DOCTYPE html>
<html>
<head>
<title>Our title</title>
<style>
#dId-1{
width: 30%;
height: 30%;
text-align:center;
padding: 20px;
margin: 10px;
border: 2px solid green;
font-size: 20px;
}
#dId-2{
width: 30%;
height: 30%;
text-align:center;
margin: 10px;
border: 2px solid green;
padding: 20px;
font-size: 20px;
}
.b1, .b2, .b3, .b4, .b5, .s1, .s2, .s3, .s4, .s5{
width:150px;
height:30px;
font-size: 20px;
}
</style>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.3.1/jquery.min.js">
</script>
<script>
$(document).ready(function(){
$(".b1").click(function(){
$(".p1").slideUp();
});
$(".b2").click(function(){
$(".p2").slideUp();
});
$(".b3").click(function(){
$(".p3").slideUp();
});
$(".b4").click(function(){
$(".p4").slideUp();
});
$(".b5").click(function(){
$(".p5").slideUp();
});
});
</script>
</head>
<body>
<div id="dId-1">
<p class="p1">This is Paragraph1 P1</p>
<p class="p2">This is Paragraph2 P2</p>
<p class="p3">This is Paragraph3 P3</p>
<p class="p4">This is Paragraph4 P4</p>
<p class="p5">This is Paragraph5 P5</p>
</div>
<div id="dId-2">
<p>
<button class="b1">SlideUp</button> SlideUp P1
</p>
<p>
<button class="b2">SlideUp</button> SlideUp P2
</p>
<p>
<button class="b3">SlideUp</button> SlideUp P3
</p>
<p>
<button class="b4">SlideUp</button> SlideUp P4
</p>
<p>
<button class="b5">SlideUp</button> SlideUp P5
</p>
<div>
</body>
</html>
Output :
1. Before slideUp() method is called.
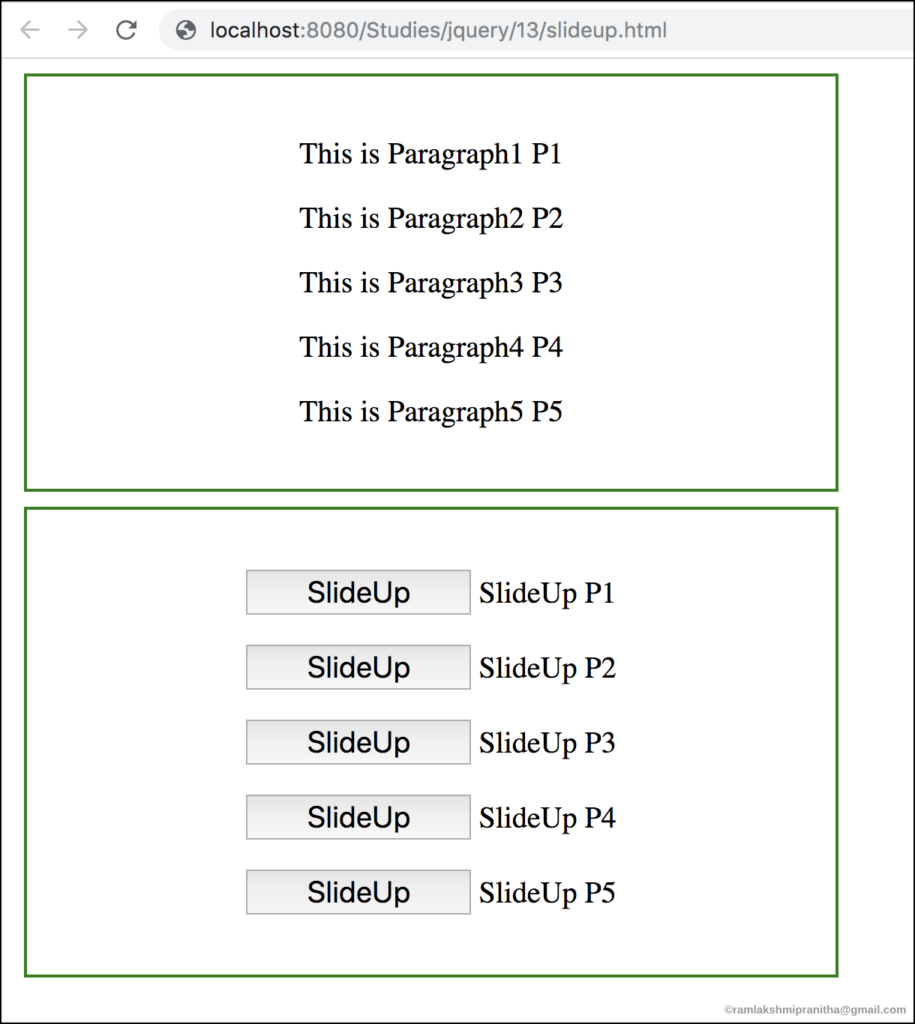
2. After slideUp() method is called.

2. slideUp() method called with callback function
Example :
<!DOCTYPE html>
<html>
<head>
<title>Our title</title>
<style>
#dId-1{
width: 30%;
height: 30%;
text-align:center;
padding: 20px;
margin: 10px;
border: 2px solid green;
font-size: 20px;
}
#dId-2{
width: 30%;
height: 30%;
text-align:center;
margin: 10px;
border: 2px solid green;
padding: 20px;
font-size: 20px;
}
.b1, .b2, .b3, .b4, .b5, .s1, .s2, .s3, .s4, .s5{
width:150px;
height:30px;
font-size: 20px;
}
</style>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.3.1/jquery.min.js">
</script>
<script>
$(document).ready(function(){
$(".b1").click(function(){
$(".p1").slideUp(function(){
alert("SlideUP function called successfully for P1");
});
});
$(".b2").click(function(){
$(".p2").slideUp(function(){
alert("SlideUP function called successfully for P2");
});
});
$(".b3").click(function(){
$(".p3").slideUp(function(){
alert("SlideUP function called successfully for P3");
});
});
$(".b4").click(function(){
$(".p4").slideUp(function(){
alert("SlideUP function called successfully for P4");
});
});
$(".b5").click(function(){
$(".p5").slideUp(function(){
alert("SlideUP function called successfully for P5");
});
});
});
</script>
</head>
<body>
<div id="dId-1">
<p class="p1">This is Paragraph1 P1</p>
<p class="p2">This is Paragraph2 P2</p>
<p class="p3">This is Paragraph3 P3</p>
<p class="p4">This is Paragraph4 P4</p>
<p class="p5">This is Paragraph5 P5</p>
</div>
<div id="dId-2">
<p>
<button class="b1">SlideUp</button> SlideUp P1
</p>
<p>
<button class="b2">SlideUp</button> SlideUp P2
</p>
<p>
<button class="b3">SlideUp</button> SlideUp P3
</p>
<p>
<button class="b4">SlideUp</button> SlideUp P4
</p>
<p>
<button class="b5">SlideUp</button> SlideUp P5
</p>
<div>
</body>
</html>
Output :
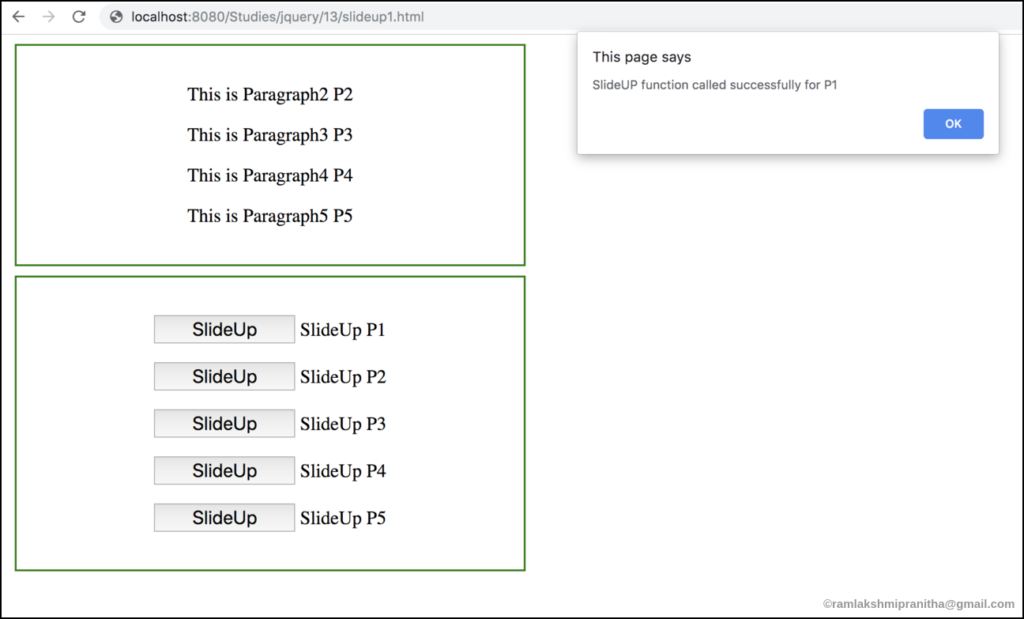