What is the jquery show() method?
JQuery inbuilt show() method is used to show the selected elements.
What is the syntax of show() ?
$(selector).show(speed,easing,callback)
selector – this is the html element.
show() – inbuilt jquery method
There are 3 params for the show() method.
1. speed
2. easing (optional)
3. callback
1. speed
This defines the speed of the show element.
By default this will be 400 milliseconds.
2. easing (optional)
This defines the speed of the element at different point of animation.
3. callback
This defines the call back function to be executed after show operation.
Jquery tutorial for beginners !!!
Click to Learn More about – online learn jquery
1. Showing elements using the show() method
Example 1 :
<!DOCTYPE html>
<html>
<head>
<title>Our title</title>
<style>
#dId-1{
width: 30%;
height: 30%;
text-align:center;
padding: 20px;
margin: 10px;
border: 2px solid green;
font-size: 20px;
}
#dId-2{
width: 30%;
height: 30%;
text-align:center;
margin: 10px;
border: 2px solid green;
padding: 20px;
font-size: 20px;
}
.b1, .b2, .b3, .b4, .b5, .s1, .s2, .s3, .s4, .s5{
width:100px;
height:30px;
font-size: 20px;
}
</style>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.3.1/jquery.min.js">
</script>
<script>
$(document).ready(function(){
$(".b1").click(function(){
$(".p1").hide();
});
$(".b2").click(function(){
$(".p2").hide();
});
$(".b3").click(function(){
$(".p3").hide();
});
$(".b4").click(function(){
$(".p4").hide();
});
$(".b5").click(function(){
$(".p5").hide();
});
$(".s1").click(function(){
$(".p1").show();
});
$(".s2").click(function(){
$(".p2").show();
});
$(".s3").click(function(){
$(".p3").show();
});
$(".s4").click(function(){
$(".p4").show();
});
$(".s5").click(function(){
$(".p5").show();
});
});
</script>
</head>
<body>
<div id="dId-1">
<p class="p1">This is Paragraph1 P1</p>
<p class="p2">This is Paragraph2 P2</p>
<p class="p3">This is Paragraph3 P3</p>
<p class="p4">This is Paragraph4 P4</p>
<p class="p5">This is Paragraph5 P5</p>
</div>
<div id="dId-2">
<p>
<button class="b1">Hide</button> Hides P1
<button class="s1">Show</button> Shows P1
</p>
<p>
<button class="b2">Hide</button> Hides P2
<button class="s2">Show</button> Shows P2
</p>
<p>
<button class="b3">Hide</button> Hides P3
<button class="s3">Show</button> Shows P3
</p>
<p>
<button class="b4">Hide</button> Hides P4
<button class="s4">Show</button> Shows P4
</p>
<p>
<button class="b5">Hide</button> Hides P5
<button class="s5">Show</button> Shows P5
</p>
<div>
</body>
</html>
Output :
1. Elements P2 and P3 are already hidden.

2. After clicking Show button
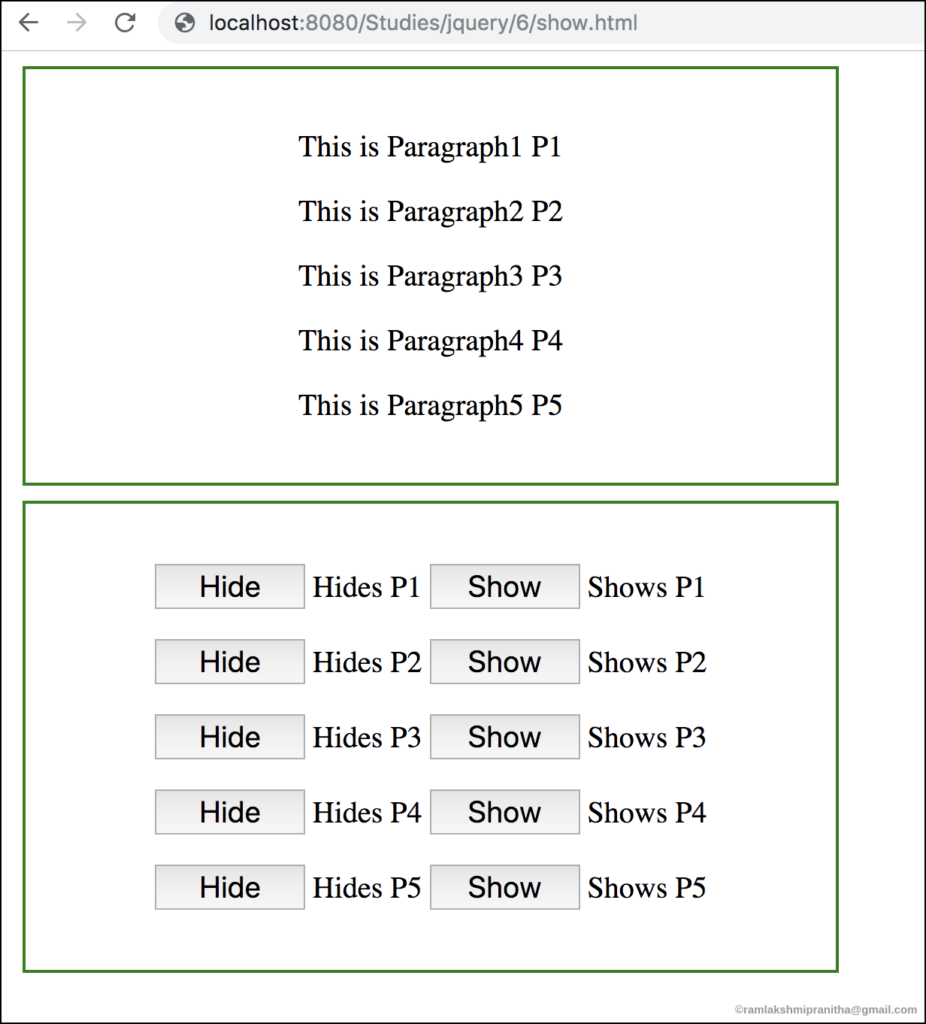
2. Passing params to the show() method.
Example 2 :
<!DOCTYPE html>
<html>
<head>
<title>Our title</title>
<style>
#dId-1{
width: 30%;
height: 30%;
text-align:center;
padding: 20px;
margin: 10px;
border: 2px solid green;
font-size: 20px;
}
#dId-2{
width: 30%;
height: 30%;
text-align:center;
margin: 10px;
border: 2px solid green;
padding: 20px;
font-size: 20px;
}
.b1, .b2, .b3, .b4, .b5, .s1, .s2, .s3, .s4, .s5{
width:100px;
height:30px;
font-size: 20px;
}
</style>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.3.1/jquery.min.js">
</script>
<script>
$(document).ready(function(){
$(".b1").click(function(){
$(".p1").hide();
});
$(".b2").click(function(){
$(".p2").hide();
});
$(".b3").click(function(){
$(".p3").hide();
});
$(".b4").click(function(){
$(".p4").hide();
});
$(".b5").click(function(){
$(".p5").hide();
});
$(".s1").click(function(){
$(".p1").show(1000, function(){
alert("P1 is shown successfully");
});
});
$(".s2").click(function(){
$(".p2").show(1000, function(){
alert("P2 is shown successfully");
});
});
$(".s3").click(function(){
$(".p3").show(1000, function(){
alert("P3 is shown successfully");
});
});
$(".s4").click(function(){
$(".p4").show(1000, function(){
alert("P4 is shown successfully");
});
});
$(".s5").click(function(){
$(".p5").show(1000, function(){
alert("P5 is shown successfully");
});
});
});
</script>
</head>
<body>
<div id="dId-1">
<p class="p1">This is Paragraph1 P1</p>
<p class="p2">This is Paragraph2 P2</p>
<p class="p3">This is Paragraph3 P3</p>
<p class="p4">This is Paragraph4 P4</p>
<p class="p5">This is Paragraph5 P5</p>
</div>
<div id="dId-2">
<p>
<button class="b1">Hide</button> Hides P1
<button class="s1">Show</button> Shows P1
</p>
<p>
<button class="b2">Hide</button> Hides P2
<button class="s2">Show</button> Shows P2
</p>
<p>
<button class="b3">Hide</button> Hides P3
<button class="s3">Show</button> Shows P3
</p>
<p>
<button class="b4">Hide</button> Hides P4
<button class="s4">Show</button> Shows P4
</p>
<p>
<button class="b5">Hide</button> Hides P5
<button class="s5">Show</button> Shows P5
</p>
<div>
</body>
</html>
Output :
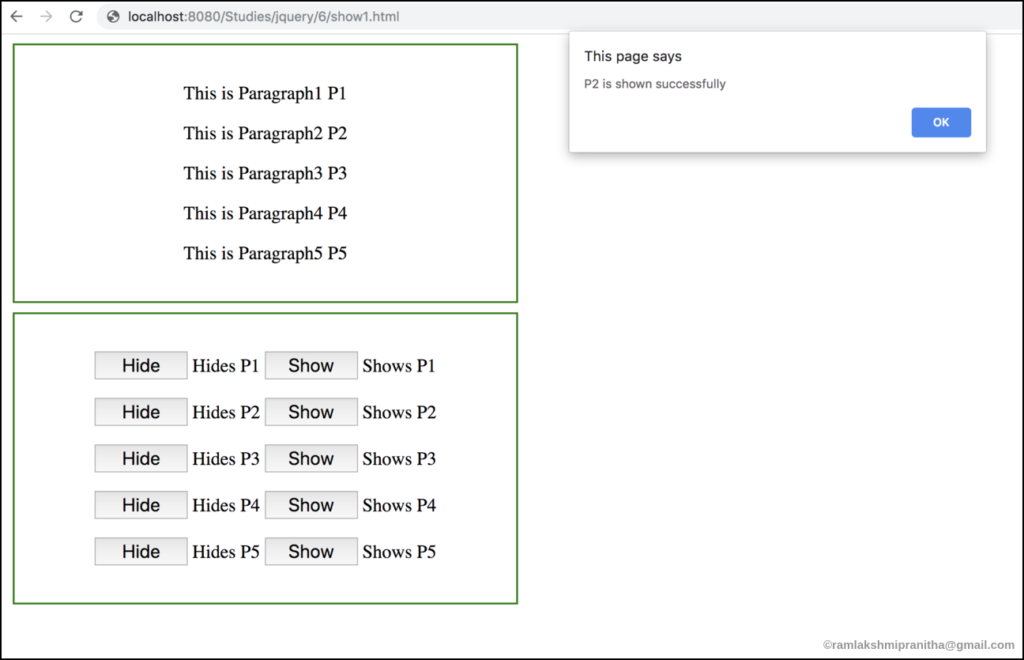