What is the jquery toggle() method?
JQuery inbuilt toggle() method is used to toggle between hide() and show() methods for the selected elements.
How does the toggle() method work?
1. show() method is executed when the element is hidden.
2. hide() method is executed when the element is visible.
What is the syntax of hide() ?
$(selector).toggle(speed,easing,callback)
selector – this is the html element.
hide() – jquery method
There are 3 optional params for toggle() method.
1. speed
2. easing
3. callback
1. speed
This defines the speed of the toggle effect.
By default this will be 400 milliseconds.
2. easing
This defines the speed of the element at different points of animation.
3. callback
This defines the call back function to be executed after toggle effect.
Jquery tutorial for beginners !!!
Click to Learn More about – online learn jquery
1. Toggle method without functional params
Example 1 :
<!DOCTYPE html>
<html>
<head>
<title>Our title</title>
<style>
#dId-1{
width: 30%;
height: 30%;
text-align:center;
padding: 20px;
margin: 10px;
border: 2px solid green;
font-size: 20px;
}
#dId-2{
width: 30%;
height: 30%;
text-align:center;
margin: 10px;
border: 2px solid green;
padding: 20px;
font-size: 20px;
}
.b1, .b2, .b3, .b4, .b5, .s1, .s2, .s3, .s4, .s5{
width:100px;
height:30px;
font-size: 20px;
}
</style>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.3.1/jquery.min.js">
</script>
<script>
$(document).ready(function(){
$(".b1").click(function(){
$(".p1").toggle();
});
$(".b2").click(function(){
$(".p2").toggle();
});
$(".b3").click(function(){
$(".p3").toggle();
});
$(".b4").click(function(){
$(".p4").toggle();
});
$(".b5").click(function(){
$(".p5").toggle();
});
});
</script>
</head>
<body>
<div id="dId-1">
<p class="p1">This is Paragraph1 P1</p>
<p class="p2">This is Paragraph2 P2</p>
<p class="p3">This is Paragraph3 P3</p>
<p class="p4">This is Paragraph4 P4</p>
<p class="p5">This is Paragraph5 P5</p>
</div>
<div id="dId-2">
<p>
<button class="b1">Toggle</button> Toggles P1
</p>
<p>
<button class="b2">Toggle</button> Toggles P2
</p>
<p>
<button class="b3">Toggle</button> Toggles P3
</p>
<p>
<button class="b4">Toggle</button> Toggles P4
</p>
<p>
<button class="b5">Toggel</button> Toggles P5
</p>
<div>
</body>
</html>
Output :
1. Toggle clicked on P2 and P2 is hidden.
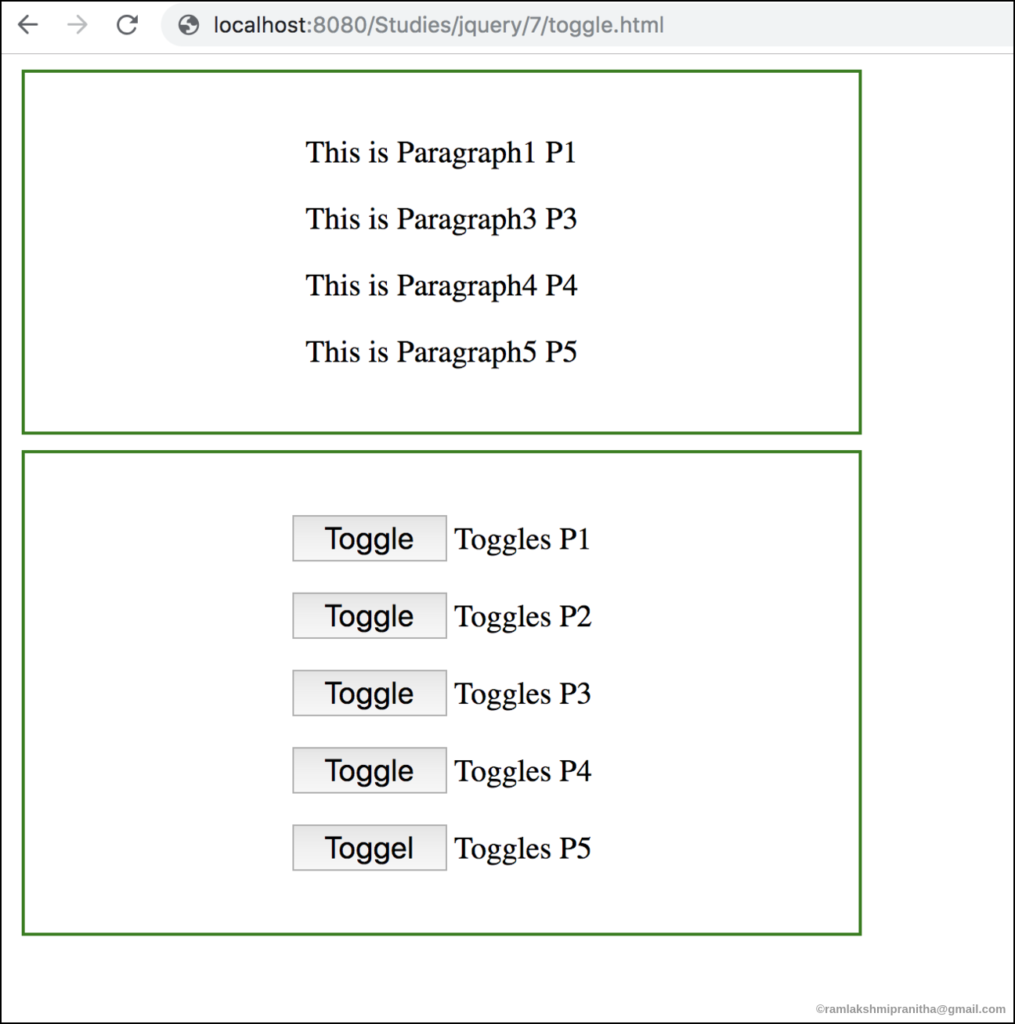
2. Again Toggle clicked on P2 and P2 is shown.
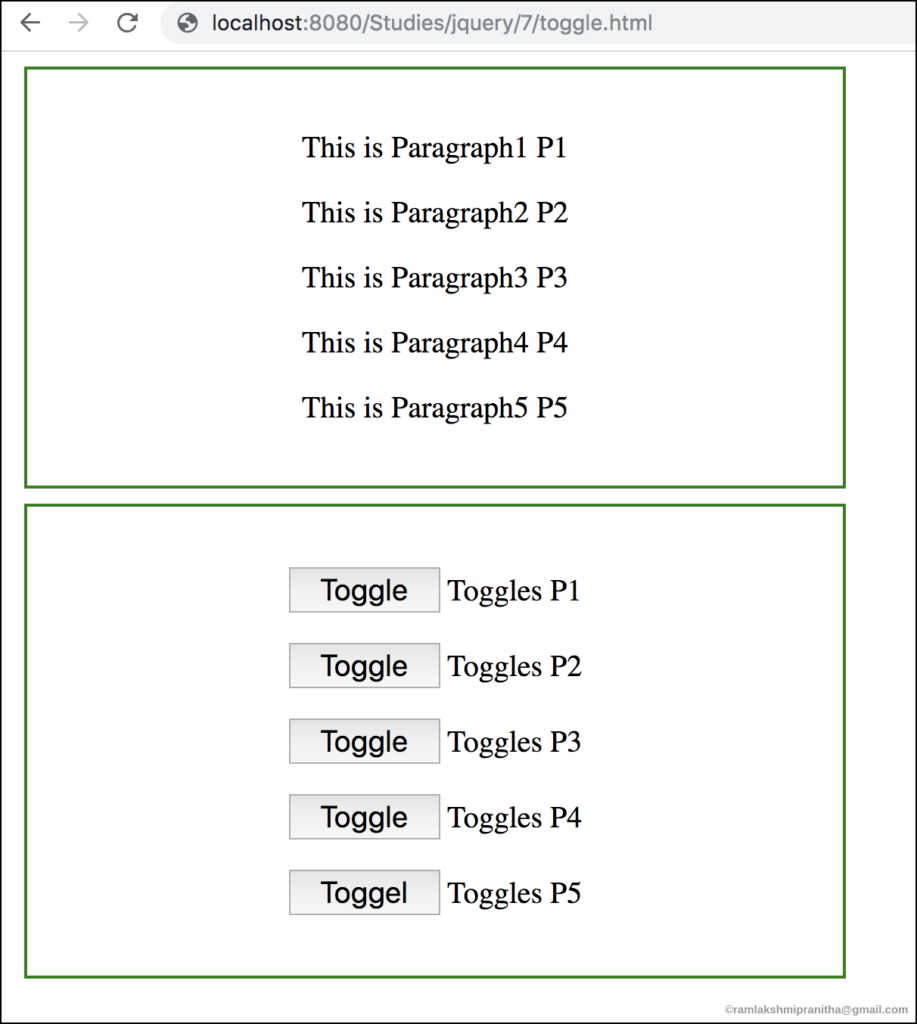
2. Toggle method with functional params
Example 2 :
<!DOCTYPE html>
<html>
<head>
<title>Our title</title>
<style>
#dId-1{
width: 30%;
height: 30%;
text-align:center;
padding: 20px;
margin: 10px;
border: 2px solid green;
font-size: 20px;
}
#dId-2{
width: 30%;
height: 30%;
text-align:center;
margin: 10px;
border: 2px solid green;
padding: 20px;
font-size: 20px;
}
.b1, .b2, .b3, .b4, .b5, .s1, .s2, .s3, .s4, .s5{
width:100px;
height:30px;
font-size: 20px;
}
</style>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.3.1/jquery.min.js">
</script>
<script>
$(document).ready(function(){
$(".b1").click(function(){
$(".p1").toggle(1000, function(){
alert("Toggle function called successfully for P1");
});
});
$(".b2").click(function(){
$(".p2").toggle(1000, function(){
alert("Toggle function called successfully for P2");
});
});
$(".b3").click(function(){
$(".p3").toggle(1000, function(){
alert("Toggle function called successfully for P3");
});
});
$(".b4").click(function(){
$(".p4").toggle(1000, function(){
alert("Toggle function called successfully for P4");
});
});
$(".b5").click(function(){
$(".p5").toggle(1000, function(){
alert("Toggle function called successfully for P4");
});
});
});
</script>
</head>
<body>
<div id="dId-1">
<p class="p1">This is Paragraph1 P1</p>
<p class="p2">This is Paragraph2 P2</p>
<p class="p3">This is Paragraph3 P3</p>
<p class="p4">This is Paragraph4 P4</p>
<p class="p5">This is Paragraph5 P5</p>
</div>
<div id="dId-2">
<p>
<button class="b1">Toggle</button> Toggles P1
</p>
<p>
<button class="b2">Toggle</button> Toggles P2
</p>
<p>
<button class="b3">Toggle</button> Toggles P3
</p>
<p>
<button class="b4">Toggle</button> Toggles P4
</p>
<p>
<button class="b5">Toggel</button> Toggles P5
</p>
<div>
</body>
</html>
Output :
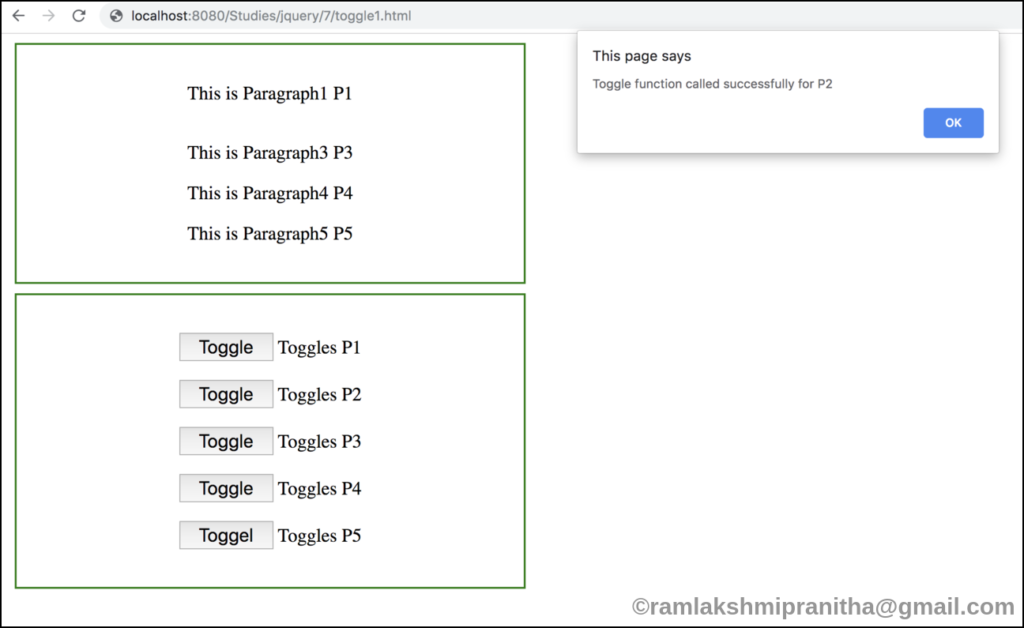